Webhook Setup
WebHook Setup
Users have the option to set up a webhook in collections and workflows in order to allow for better control over the activities carried out on the system.
To set up a webhook for either a collection or workflow; on the sidebar menu, navigate to Webhooks >> Configuration.
You can set separate webhook URLs for your Test and Live environments.
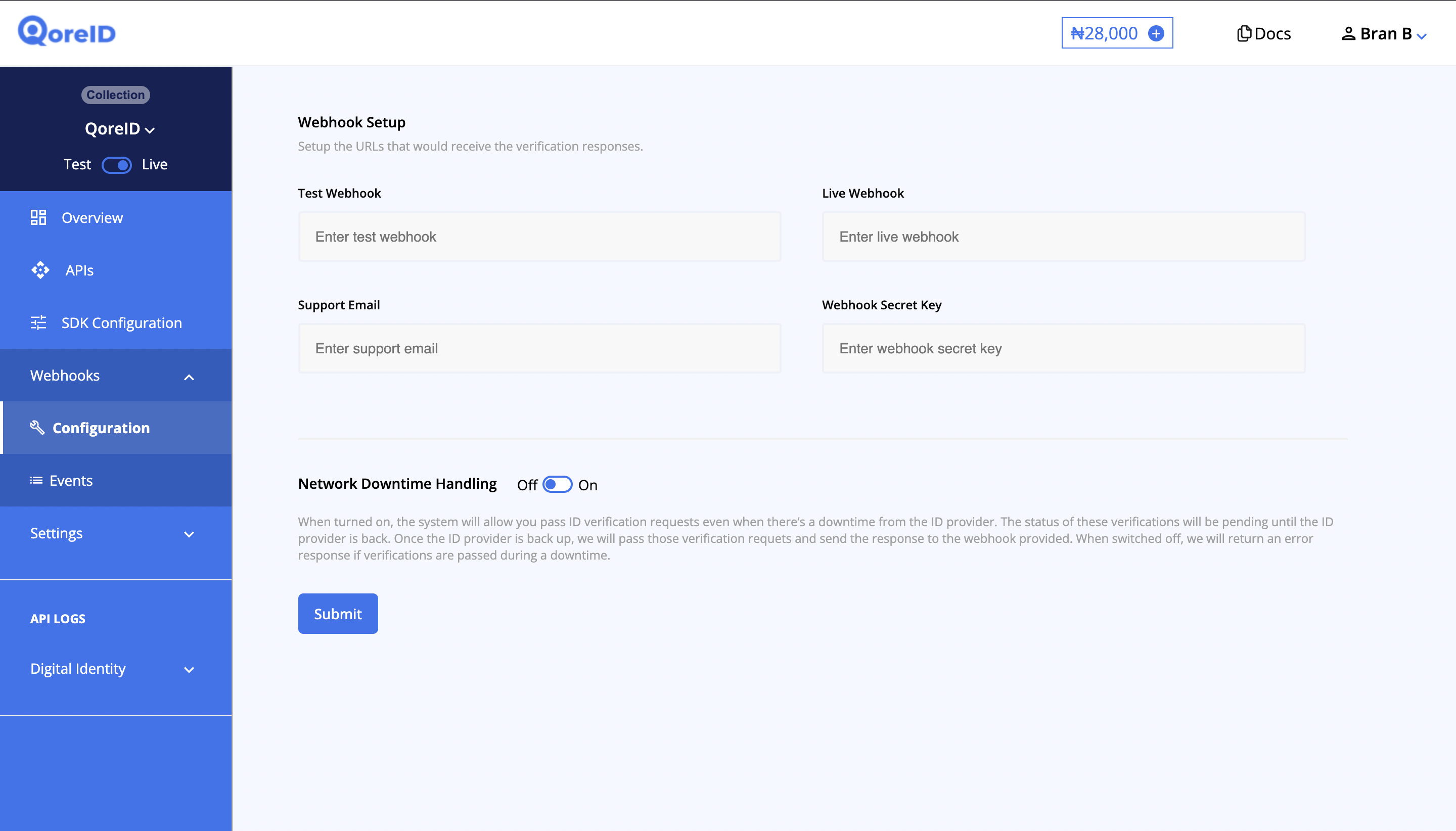
Network Downtime Handling
If this is enabled, when a realtime verification fails due to a third party provider being unavailable, your request will be retried when the third party provider is back in operation and the verification response will be sent to the webhook URL provided.
Support Email
This is the email address to be contact if your webhook endpoint is unreachable after attempting to send a webhook payload with 5 retries.
Webhook Secret Key
QoreID hashes your webhook payload using SHA512
and your provided webhook secret and adds it to the header x-verifyme-signature
as a signature with which you can validate your webhook payload.
The following shows an example webhook validation script.
const crypto = require('crypto');
const WEBHOOK_SECRET = 'your_webhook_secret';
function validateWebhookPayload(signature, payloadBody) {
let hash = crypto.createHmac('sha512', WEBHOOK_SECRET);
hash = hash.update(JSON.stringify(payloadBody)).digest('hex');
return crypto.timingSafeEqual(Buffer.from(hash), Buffer.from(signature));
}
// A sample webhook coming from QoreID
const WEBHOOK_PAYLOAD =
{
event: 'address',
event_type: 'verification_completed',
summary: {address_check: 'verified'},
status: {
status: 'verified',
state: 'complete'
},
address: {
}
};
// QoreID hashes your webhook payload and adds it to the request header
const signature = request.headers['x-verifyme-signature'];
const isValidPayload = validateWebhookPayload(signature, WEBHOOK_PAYLOAD);
using System.Security.Cryptography;
private string ToHexString(byte[] bytes)
{
var builder = new StringBuilder(bytes.Length * 2);
foreach (var b in bytes)
{
builder.AppendFormat("{0:x2}", b);
}
return builder.ToString();
}
private bool validateWebhookPayload(string body, string signature , string webhookSecret)
{
var secretBytes = Encoding.UTF8.GetBytes(webhookSecret);
var payloadBytes = Encoding.UTF8.GetBytes(body);
var hmac = new HMACSHA512(secretBytes);
var generatedHmacHashBytes = hmac.ComputeHash(payloadBytes);
return ToHexString(generatedHmacHashBytes) == signature;
}
// QoreID hashes your webhook payload and adds it to the request header
var signature = request.Headers['X-Verifyme-Signature'];
var payload = request.Body // webhook payload
var webhoookSecret = "your_webhook_secret"
var isValidPayload = validateWebhookPayload(payload, signature, webhoookSecret);
Updated over 1 year ago
Proceed to your QoreID dashboard to begin setting up your collections and workflows webhooks